Mastering Python Logging for Effective Application Monitoring
Written on
Chapter 1 Understanding Python Logging
Python's logging framework is an essential resource for creating log entries and overseeing log files. Logging entails the process of documenting messages generated by software applications, which can be pivotal in tracking and analyzing application performance. These messages assist in monitoring system efficiency, diagnosing errors, and comprehending user interactions. The primary goal of logging is to maintain a comprehensive record of events during application execution, aiding developers in identifying and rectifying issues, thereby enhancing the application's overall reliability.
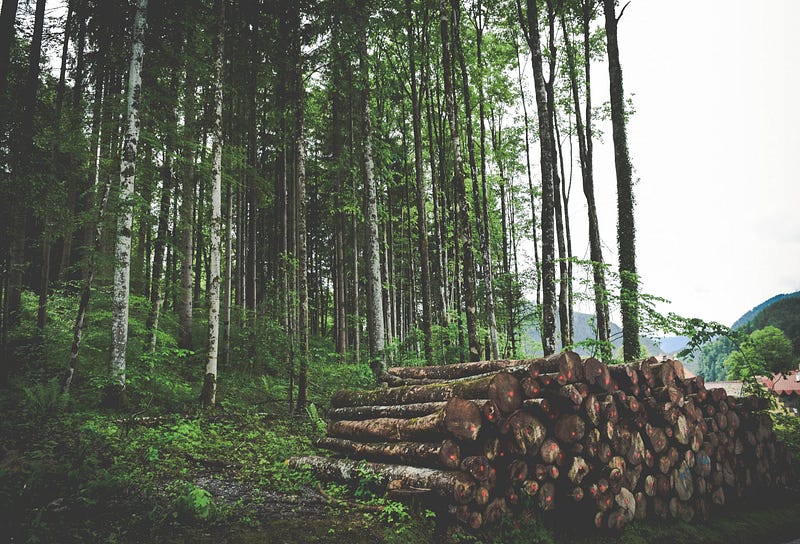
Logging Levels Explained
Python's logging framework offers multiple levels to classify log messages by their importance. The levels, listed in increasing order of severity, include:
- DEBUG: In-depth information useful for debugging.
- INFO: General updates about the application's status.
- WARNING: Alerts that something unexpected occurred, indicating potential issues ahead.
- ERROR: Errors that may necessitate attention.
- CRITICAL: Severe errors that could lead to application termination.
Configuring Logging in Python
To set up logging in Python, one must establish a logger object representing the application or module intended for logging. This object facilitates the definition of logging parameters and directs log messages to the suitable handlers. Below is a snippet illustrating how to create a logger object:
import logging
logger = logging.getLogger(__name__)
This line generates a logger named after the current module. Alternatively, a logger can be designated a different name to denote a specific application or component.
Logging Handlers Overview
Log messages are dispatched from the logger object to logging handlers, which manage the destination of these messages. Python supports several handler types, including:
- StreamHandler: Directs log messages to a stream (like stdout or stderr).
- FileHandler: Outputs log messages to a file.
- RotatingFileHandler: Writes log messages to a file that rotates based on size or time.
- SMTPHandler: Sends log messages via email.
Here’s an example of how to implement a FileHandler:
file_handler = logging.FileHandler('app.log')
file_handler.setLevel(logging.DEBUG)
logger.addHandler(file_handler)
This code snippet creates a FileHandler that records log messages in a file named 'app.log'. The setLevel() method establishes the minimum logging level for this handler, while addHandler() links the handler to the logger object.
Logging Messages in Practice
After configuring the logger and integrating the relevant handlers, you can start logging messages. For instance:
logger.info('Application started')
This logs an INFO level entry with the message "Application started." All logging levels defined by the framework can be utilized to categorize your log messages effectively.
The first video titled "Python Logging Demystified: Part 1 - Concepts" delves into foundational aspects of Python's logging module, offering a clear overview of its functionalities.
Conclusion
The logging module in Python serves as a vital tool for creating log entries and managing log files. By classifying log messages according to their severity, developers can swiftly pinpoint issues and resolve errors. Moreover, by configuring logging handlers, control over where messages are recorded and their formatting can be achieved. Effective logging practices provide insights into application behavior, ultimately fostering improved reliability.
The second video, "Python Logging Demystified: Part 2 - Coding," showcases practical coding examples that illustrate the application of logging in real-world scenarios.