Understanding Linked Lists: A Comprehensive Guide for Beginners
Written on
Chapter 1: Introduction to Linked Lists
Linked lists are categorized as non-primitive and linear data structures. They are deemed non-primitive because they are constructed using primitive data types, and linear due to the linear arrangement of data within them.
Table of Contents
- Self-Referential Structures
- What Are Linked Lists?
- Advantages of Linked Lists
- Disadvantages of Linked Lists
- Applications of Linked Lists
- Linked Lists vs. Arrays
- Time Complexity of Linked Lists
- Stay Connected
To grasp the concept of linked lists, it’s essential to first delve into self-referential data structures.
Section 1.1: Self-Referential Structures
Self-referential structures consist of one or more pointers that refer to instances of the same structure as their members. This implies that these structures include pointer members that store the address of other instances of the same type, thus allowing for the linking of an unspecified number of such structures.
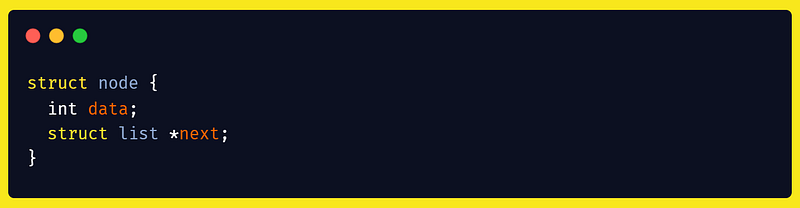
In a visual representation, it can be depicted as follows:
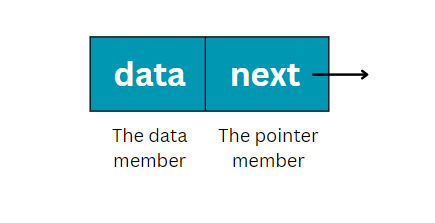
In this illustration, the data member holds the actual data while the next pointer contains the address of the subsequent structure of the same type. Linked lists exemplify self-referential data structures, where a pointer in one node points to the next node.
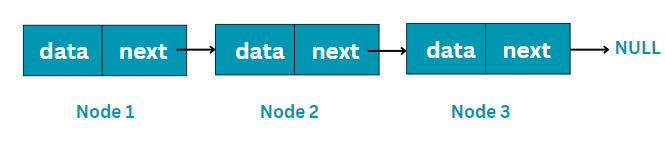
Section 1.2: What Are Linked Lists?
A linked list is a collection of nodes that are stored randomly in memory. This structure allows for dynamic management of data elements, in contrast to arrays, where data items are kept in contiguous memory locations. In linked lists, elements exist in various locations and are connected through pointers.
What is a Node?
A node generally comprises two parts: data (or link) and a pointer (or next). The data field contains the actual data stored at that specific location, while the pointer field holds the address of the following node in memory.
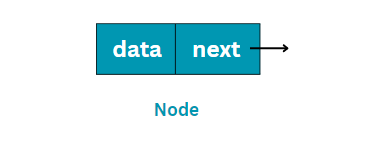
For the last node within a linked list, the pointer will be null since there are no additional nodes to reference. The head pointer indicates the first node of the linked list.
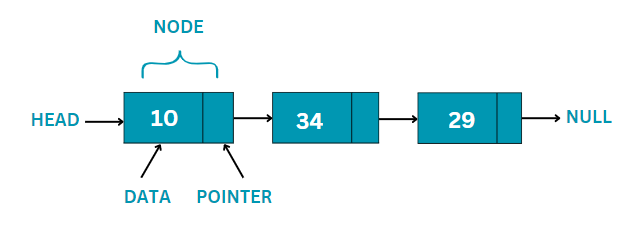
Section 1.3: Why Choose Linked Lists?
While arrays are widely utilized and offer multiple functionalities, they come with significant limitations. Arrays are static, meaning their size is fixed at creation, and this rigidity can lead to inefficiencies. For instance, if memory is limited or if the array size is excessively large, it can result in memory shortages and program crashes.
Inserting a new element into an array is costly, as it necessitates shifting existing elements to accommodate the new entry. Similarly, deletions can be cumbersome unless special techniques are employed.
Linked lists address these limitations in several ways:
- Dynamic Structure: Unlike arrays, linked lists are dynamic, allowing their size to be adjusted as needed.
- Efficient Memory Use: Linked lists dynamically utilize memory, mitigating waste.
- Simplified Insertion/Deletion: Adding or removing elements is straightforward since no data shifting is required; only the pointers need updating.
- Versatile Applications: Like arrays, linked lists can serve as the foundation for other data structures such as stacks, queues, graphs, and hash maps.
The first video titled "Linked Lists 101: Your Essential Guide to Data Structures for Beginners" offers a foundational overview of linked lists and their significance in data structures.
Section 1.4: Representation of Linked Lists
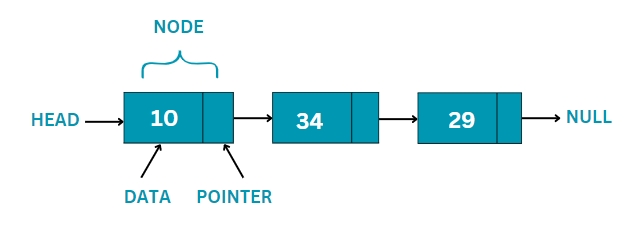
A linked list comprises nodes, each pointing to the next. Each node contains a data item and a reference to the subsequent node. The head pointer points to the first node in the list, while the data field holds the node's value. The pointer field references the next node, which is null for the final element.
Advantages of Linked Lists
- Dynamic Sizing: Linked lists adjust their size dynamically, unlike static arrays.
- Ease of Insertion/Deletion: Adding or removing nodes is simple and does not require shifting elements.
- Memory Efficiency: They utilize only the necessary memory for their data elements.
- Optimal Time Complexity: Inserting a new node at the beginning takes constant time (O(1)), unlike arrays where it takes linear time (O(n)).
Disadvantages of Linked Lists
- No Random Access: Accessing elements requires sequential traversal.
- Extra Memory Requirements: More memory is needed for pointers.
- No Locality of Reference: Linked lists do not benefit from memory locality, making them less cache-friendly.
- Complex Sorting: Sorting linked lists can be complicated due to the need for pointer manipulation.
Applications
Linked lists serve numerous purposes, including implementing various data structures, handling dynamic memory allocation, and managing processes in operating systems. They are also employed in everyday applications, such as music players and web browsers, where elements are frequently navigated.
The second video titled "Learn Linked Lists in 13 Minutes" provides a concise yet thorough explanation of linked lists and their applications in programming.
Chapter 2: Comparing Linked Lists and Arrays
The distinctions between linked lists and arrays are notable:
- Arrays hold data in contiguous memory, while linked lists store elements in non-contiguous locations.
- Arrays are static, with fixed sizes, whereas linked lists can expand or contract as required.
- Memory allocation for arrays occurs at compile time; linked lists allocate memory at runtime.
- Linked lists require additional memory for pointers, which can be significant with large datasets.
In conclusion, while linked lists may consume more memory, they offer flexibility that often results in more efficient memory usage overall, particularly for dynamic applications.
Linked List Time Complexity
The time complexity for linked list operations varies:
- Searching requires O(n) time due to sequential access.
- Insertion at the start is O(1).
- Insertion at the end and at specific positions requires O(n).
- Deletion operations also vary, with O(1) for the head and O(n) for others.
Stay Connected
Feel free to connect on Twitter! I also write about writing, productivity, and self-improvement. If these topics interest you, check out my Medium profile and subscribe to my newsletter for a free e-book, "Beginner’s Guide to Writing on Medium."
Have a great day!